mirror of
https://codeberg.org/davrot/forgejo.git
synced 2025-07-05 19:00:01 +02:00
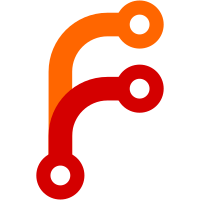
This is my take to fix #6078 Should also resolve #6111 As far as I can tell, Forgejo uses only a subset of the relative-time functionality, and as far as I can see, this subset can be implemented using browser built-in date conversion and arithmetic. So I wrote a JavaScript to format the relative-time element accordingly, and a Go binding to generate the translated elements. This is my first time writing Go code, and my first time coding for a large-scale server application, so please tell me if I'm doing something wrong, or if the whole approach is not acceptable. --- Screenshot: Localized times in Low German  Screenshot: The same with Forgejo in English  --- ## Checklist The [contributor guide](https://forgejo.org/docs/next/contributor/) contains information that will be helpful to first time contributors. There also are a few [conditions for merging Pull Requests in Forgejo repositories](https://codeberg.org/forgejo/governance/src/branch/main/PullRequestsAgreement.md). You are also welcome to join the [Forgejo development chatroom](https://matrix.to/#/#forgejo-development:matrix.org). ### Tests - I added test coverage for Go changes... - [x] in their respective `*_test.go` for unit tests. - [ ] in the `tests/integration` directory if it involves interactions with a live Forgejo server. - I added test coverage for JavaScript changes... - [x] in `web_src/js/*.test.js` if it can be unit tested. - [ ] in `tests/e2e/*.test.e2e.js` if it requires interactions with a live Forgejo server (see also the [developer guide for JavaScript testing](https://codeberg.org/forgejo/forgejo/src/branch/forgejo/tests/e2e/README.md#end-to-end-tests)). ### Documentation - [ ] I created a pull request [to the documentation](https://codeberg.org/forgejo/docs) to explain to Forgejo users how to use this change. - [x] I did not document these changes and I do not expect someone else to do it. ### Release notes - [x] I do not want this change to show in the release notes. - [ ] I want the title to show in the release notes with a link to this pull request. - [ ] I want the content of the `release-notes/<pull request number>.md` to be be used for the release notes instead of the title. Reviewed-on: https://codeberg.org/forgejo/forgejo/pulls/6154 Reviewed-by: 0ko <0ko@noreply.codeberg.org> Reviewed-by: Michael Kriese <michael.kriese@gmx.de> Co-authored-by: Benedikt Straub <benedikt-straub@web.de> Co-committed-by: Benedikt Straub <benedikt-straub@web.de>
212 lines
9 KiB
JavaScript
212 lines
9 KiB
JavaScript
import {DoUpdateRelativeTime, HALF_MINUTE, ONE_MINUTE, ONE_HOUR, ONE_DAY} from './relative-time.js';
|
|
|
|
test('CalculateRelativeTimes', () => {
|
|
window.config.pageData.PLURAL_RULE_LANG = 0;
|
|
window.config.pageData.DATETIMESTRINGS = {
|
|
'FUTURE': 'in future',
|
|
'NOW': 'now',
|
|
'relativetime.1day': 'yesterday',
|
|
'relativetime.1week': 'last week',
|
|
'relativetime.1month': 'last month',
|
|
'relativetime.1year': 'last year',
|
|
'relativetime.2days': 'two days ago',
|
|
'relativetime.2weeks': 'two weeks ago',
|
|
'relativetime.2months': 'two months ago',
|
|
'relativetime.2years': 'two years ago',
|
|
};
|
|
window.config.pageData.PLURALSTRINGS_LANG = {
|
|
'relativetime.mins': ['%d minute ago', '%d minutes ago'],
|
|
'relativetime.hours': ['%d hour ago', '%d hours ago'],
|
|
'relativetime.days': ['%d day ago', '%d days ago'],
|
|
'relativetime.weeks': ['%d week ago', '%d weeks ago'],
|
|
'relativetime.months': ['%d month ago', '%d months ago'],
|
|
'relativetime.years': ['%d year ago', '%d years ago'],
|
|
};
|
|
const mock = document.createElement('relative-time');
|
|
|
|
const now = Date.parse('2024-10-27T04:05:30+01:00'); // One hour after DST switchover, CET.
|
|
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(null);
|
|
expect(mock.textContent).toEqual('');
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T04:06:40+01:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(70000);
|
|
expect(mock.textContent).toEqual('in future');
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T04:05:10+01:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(HALF_MINUTE);
|
|
expect(mock.textContent).toEqual('now');
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T04:04:30+01:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_MINUTE);
|
|
expect(mock.textContent).toEqual('1 minute ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T04:04:00+01:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_MINUTE);
|
|
expect(mock.textContent).toEqual('1 minute ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T04:03:20+01:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_MINUTE);
|
|
expect(mock.textContent).toEqual('2 minutes ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T04:00:00+01:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_MINUTE);
|
|
expect(mock.textContent).toEqual('5 minutes ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T03:59:30+01:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_MINUTE);
|
|
expect(mock.textContent).toEqual('6 minutes ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T03:01:00+01:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_HOUR);
|
|
expect(mock.textContent).toEqual('1 hour ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T01:00:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_HOUR);
|
|
expect(mock.textContent).toEqual('4 hours ago'); // This tests DST switchover
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T00:01:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_HOUR);
|
|
expect(mock.textContent).toEqual('5 hours ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-26T22:00:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_HOUR);
|
|
expect(mock.textContent).toEqual('7 hours ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-26T05:08:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_HOUR);
|
|
expect(mock.textContent).toEqual('23 hours ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-26T04:00:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('yesterday');
|
|
|
|
mock.setAttribute('datetime', '2024-10-25T01:00:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('two days ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-21T01:00:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('6 days ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-20T01:00:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('last week');
|
|
|
|
mock.setAttribute('datetime', '2024-10-14T01:00:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('last week');
|
|
|
|
mock.setAttribute('datetime', '2024-10-13T01:00:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('two weeks ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-06T01:00:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('3 weeks ago');
|
|
|
|
mock.setAttribute('datetime', '2024-09-25T01:00:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('last month');
|
|
|
|
mock.setAttribute('datetime', '2024-08-30T01:00:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('last month');
|
|
|
|
mock.setAttribute('datetime', '2024-07-30T01:00:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('two months ago');
|
|
|
|
mock.setAttribute('datetime', '2024-05-30T01:00:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('4 months ago');
|
|
|
|
mock.setAttribute('datetime', '2024-03-01T01:00:00+01:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('7 months ago');
|
|
|
|
mock.setAttribute('datetime', '2024-02-29T01:00:00+01:00'); // Leap day handling
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('7 months ago');
|
|
|
|
mock.setAttribute('datetime', '2024-02-27T01:00:00-03:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('7 months ago');
|
|
|
|
mock.setAttribute('datetime', '2024-02-27T01:00:00+01:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('8 months ago');
|
|
|
|
mock.setAttribute('datetime', '2023-11-15T01:00:00+03:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('11 months ago');
|
|
|
|
mock.setAttribute('datetime', '2023-10-20T01:00:00+08:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('last year');
|
|
|
|
mock.setAttribute('datetime', '2022-10-30T01:00:00-05:30');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('last year');
|
|
|
|
mock.setAttribute('datetime', '2022-10-20T01:00:00+10:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('two years ago');
|
|
|
|
mock.setAttribute('datetime', '2021-10-20T01:00:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('3 years ago');
|
|
|
|
mock.setAttribute('datetime', '2014-10-20T01:00:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_DAY);
|
|
expect(mock.textContent).toEqual('10 years ago');
|
|
|
|
// Timezone tests
|
|
mock.setAttribute('datetime', '2024-10-27T01:05:30-05:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(3 * ONE_HOUR);
|
|
expect(mock.textContent).toEqual('in future');
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T05:05:25+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(HALF_MINUTE);
|
|
expect(mock.textContent).toEqual('now');
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T05:04:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_MINUTE);
|
|
expect(mock.textContent).toEqual('1 minute ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T05:02:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_MINUTE);
|
|
expect(mock.textContent).toEqual('3 minutes ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T04:06:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_MINUTE);
|
|
expect(mock.textContent).toEqual('59 minutes ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T04:05:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_HOUR);
|
|
expect(mock.textContent).toEqual('1 hour ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T01:00:00+02:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_HOUR);
|
|
expect(mock.textContent).toEqual('4 hours ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T01:00:00+04:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_HOUR);
|
|
expect(mock.textContent).toEqual('6 hours ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T01:00:00+10:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_HOUR);
|
|
expect(mock.textContent).toEqual('12 hours ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-27T01:00:00Z');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_HOUR);
|
|
expect(mock.textContent).toEqual('2 hours ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-26T15:00:00-01:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_HOUR);
|
|
expect(mock.textContent).toEqual('11 hours ago');
|
|
|
|
mock.setAttribute('datetime', '2024-10-25T19:00:00-11:00');
|
|
expect(DoUpdateRelativeTime(mock, now)).toEqual(ONE_HOUR);
|
|
expect(mock.textContent).toEqual('21 hours ago');
|
|
});
|